Project Mapbox + Turf.js on Rails Mapbox API using Turf.js within a Ruby on Rails Application
Mapbox + Turf.js on Rails is a mapping & geospatial application that geocodes, maps & calculates locations with Mapbox GL API, Turf.js & Geokit within a Ruby on Rails application. Use it in your new or existing Rails app to provide rich mapping & data visualization features.
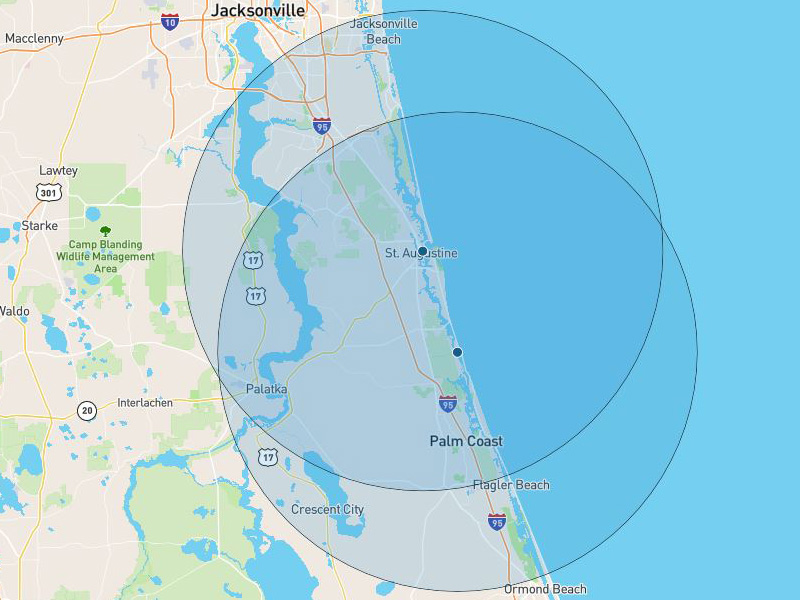
Mapbox + Turf.js on Rails was originally based off the hard work in Rui Freitas' Mapbox Clusters repo. His Model => GeoJSON work was extremely helpful in building the dataset Mapbox & Turf.js needed to populate a Mapbox map. Mapbox API is a great mapping & location tool to develop with. However, plotting a geospatial accurate radius can be challenging and require quite a bit of tile & zoom modifications to get it to work. Turf.js is a geospatial tool that makes it easy to calculate GeoJSON based polygons among a whole bunch of other helpful tools. Integrating Turf.js into a Mapbox API on Rails was something that I hadn't been able to find, so I built a solution.
const initMapbox = () => {
const mapElement = document.getElementById('map');
if (mapElement) {
mapboxgl.accessToken = mapElement.dataset.mapboxApiKey;
const map = new mapboxgl.Map({
container: 'map',
style: 'mapbox://styles/mapbox/streets-v10'
});
map.on('load', function() {
const offices = JSON.parse(mapElement.dataset.offices);
var radius = 30;
var options = {
steps: 80,
units: 'miles'
};
var circles = offices.coordinates.map(e =>
turf.circle(e, radius, options)
);
const office_circles = {
'type': 'FeatureCollection',
'features': circles
};
map.addSource('offices', {
type: 'geojson',
data: offices
});
map.addSource('office_circles', {
type: 'geojson',
data: office_circles
});
Mapbox builds the map layers and elements within the Mapbox.js file. The model data attributres are parsed as GeoJSON and passed from the controller to the javascript file. Passing the coordinates to Turf.js allowed the turf.cirle
method to calculate the radius on the fly.
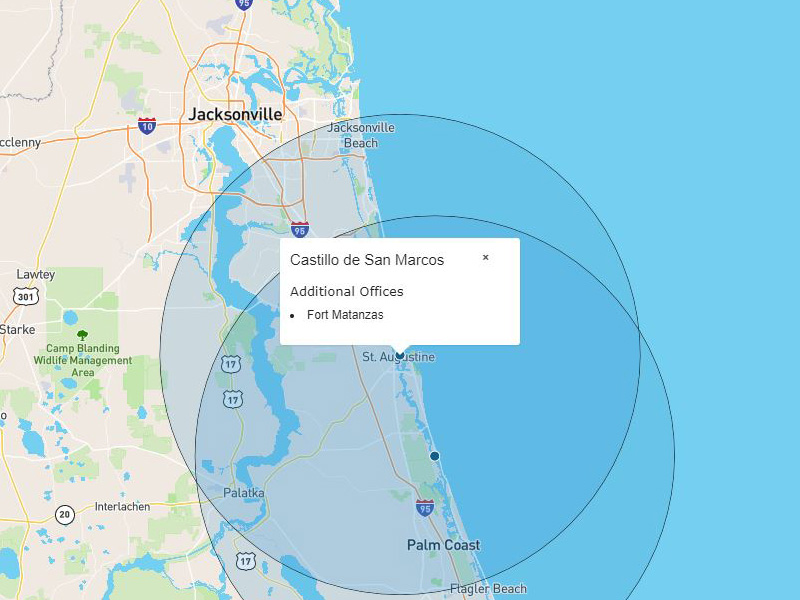
within
MethodAnother feature the application has is its ability to query offices within a defined mile radius and present the results of that data query. I was able to use this block in the models/office.rb
to call that method.
def within_radius
Office.within(30, :origin => coordinates)
end
I really enjoyed working within the Mapbox API ecosystem and being able to utilize Turf.js without much trouble expands the flexibility of the API. I plan on working these tools into future projects.